What is an API?
API stands for Application Program Interface. An API is an intermediate layer in a software system that is responsible for the data communication between the data source and the Graphical User Interface (GUI) that user’s see. In other words, the API is the business layer of the software that creates a connection between the presentation layer and the data layer.
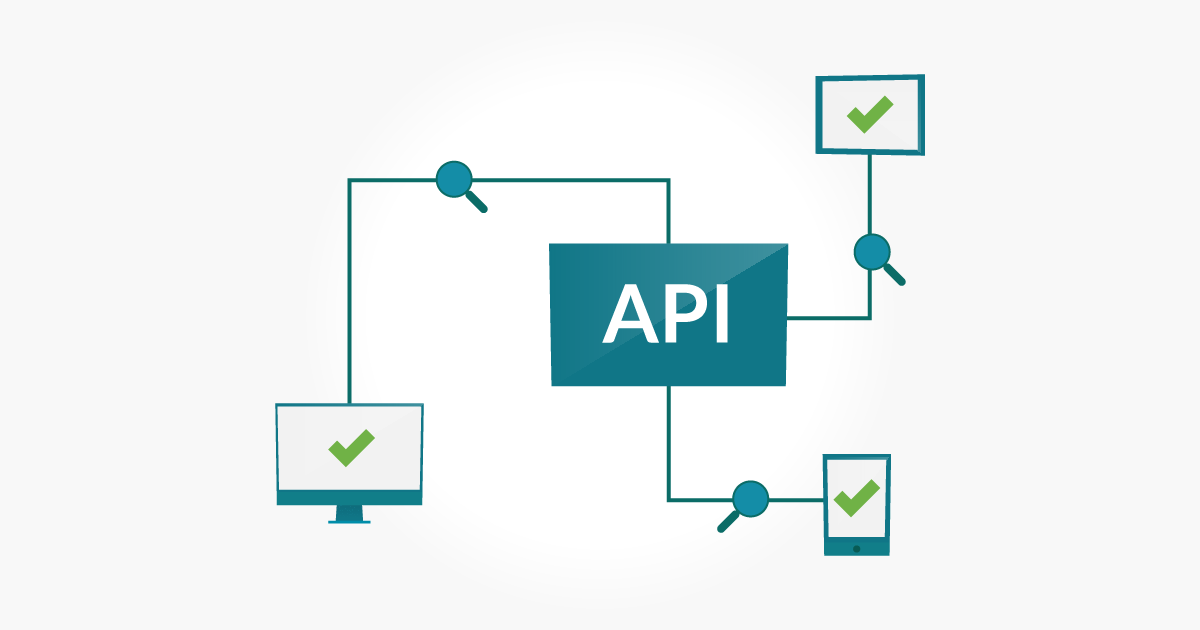
API testing focuses on what’s known as the business layer of the application, meaning the testing methods will be entirely different compared to the standard GUI testing. So instead of using keyboard strokes and mouse clicks as standard input, in API testing we may need to use some other testing patterns, use some testing tools or our own code in order to examine these APIs under test. You need to validate the responses (output) from these APIs in order to verify that they are working, or not. To know what to look for, you will need be aware of the response that an API generates.
An API response will be:
- A status message/Boolean value (for example, success/error, or true/false etc.) which will display the status of the API call. It will also act as a flag (true/false), on which the Presentation layer or the Database layer will be then updated.
- A set of data which is to be passed on to a subsequent API, or to the GUI or to the Database.
What to Test for in APIs?
- Functional: This is where you look for the API response based on the input provided. Check whether the actual response matches the response expected.
- Performance: Here, you need to be aware of the API’s response time. Occasionally, it takes a long time to get a response from the API. This can be due to a performance issue related to the API’s design.
- Security: Checking whether any sensitive data passing onto the API has been encrypted, or not, is part of this testing. For example, you may want to check an API that is responsible for generating a dashboard report on a home page. In order to access the dashboard API, you may need a token that would have been generated as a response from the login API previously run. This token should be in encrypted format. You can also check if there is any HTTPS encryption in place.
- Reliability: You can check whether the API gives you prompt response everytime you test for different configurations (for example, different environments, various user logins etc.) You can check if the output returns any exception handling errors, timeout errors etc. which will point to the reliability of the API. Part of reliability testing you can also check whether the response data is structured properly. Usually API response will be structured in JSON or XML format.
- Negative Testing: Here the aim is to provide invalid input data to the API and check how the output data behaves. API should handle the errors properly. It should provide valid, meaningful error messages for every negative input conditions. You can also test the behaviour of the API if empty input data is used for some arguments. You can also look out for any unused flags, missing/duplicate output values for different input data.
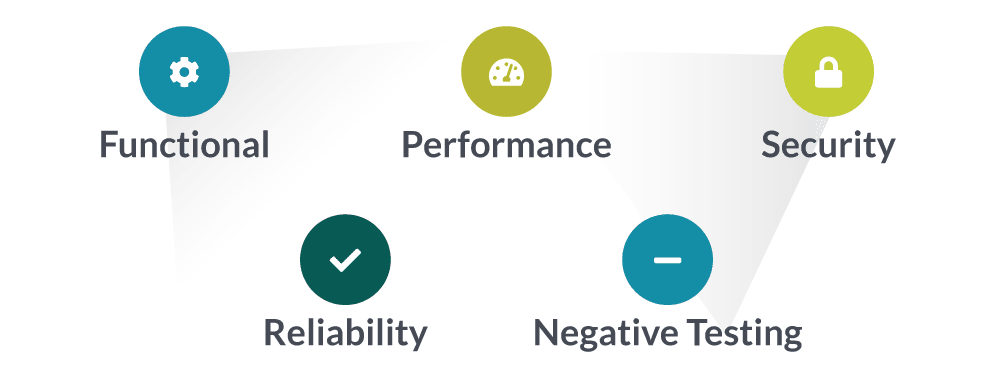
What does an API test case look like?
The below components should be included in a API test case document.
Test Steps
The API URL
This is the HTTP request for invoking a particular API. Take, for example, an API for login functionality. If its URL structure is Mobile/User/Login and your domain URL is http://domain.com, then the API URL to invoke the API from browser/tools will be http://domain.com/Mobile/User/Login
HTTP Method
In the case of RESTful APIs, they use HTTP methods to classify the APIs based on the type of call made to the server. For example, POST, GET etc. The POST method sends data to the server while the GET method fetches the data back from the server.
Payload
This defines the structure, or the model of input data, which is to be supplied to the API. For example, if we take the above Login API we can use the below data structure:
{
username:string
password:string
device_id:string
object_id:string
device_token:string
mobile_os:string
app_language:string
}
Request Sample Data
Request Sample contains the actual input data which is passed on to the API as a payload. You can have as many test cases as you want based on this sample data. For the above login scenario, the sample data might look like this:
{
“device_id”:”F3649737-B25D-43BA-A212-71192″,
“object_id”:””,
“device_token”:”f4icqBpC04k:APA91bFFYp8MKaetZKiAJ,
“mobile_os”:”iOS”,
“App_language”:”en”
}
Expected result
Response Code
This represents the response code of the API request. 200 OK should be the response Code of a successful API request. There are other Response codes such as 400 Bad request, 401 Unauthorized, 403 Forbidden, 404 Not found, 500 Internal Server Error etc. It would be useful if you could note down the Response Code of the API from the output console of the API testing tool.
Response Result Message
For each input, there might be different API output success messages. You need to figure out the corresponding response messages and document them in your test cases. Some common messages for Login API will be: SUCCESS, INACTIVE_ACCOUNT, INVALID_PASSWORD, USER_NOT_FOUND, INVALID_DEVICE_ID, ERROR
Response Result Sample
This is the output data for each of the input data combinations. You will need this data to be able to validate against the actual output from the API results. A sample for a successful Login operation is given below:
{
“result”: “SUCCESS”,
“data”: {
“id”: 7093,
“company_id”: 0,
“customer_id”: “181055033”,
“user_type”: 0,
“username”: “user@company.com”,
“first_name”: “Test”,
“last_name”: “User”,
“app_language”: “en”,
“mobile_os”: “iOS”,
“email”: “user@company.com”,
“phone”: “917837322”,
“secondary_phone”: “0”,
“address”: “”,
“created_at”: “2018-10-01”,
“updated_at”: “2018-10-01”,
“last_login”: “2018-10-01”,
}}
Tips for API Testing
- Understand what each API is used for in the application. Without understanding the use of a particular API, it will be difficult to document sufficient test cases for it.
- When writing test cases for different input conditions, make use of testing techniques such as Boundary Value Analysis and Equivalence Class Partitioning.
- Properly document the input parameters and response of the API for each of the test cases so that testing can be carried out in a structured way. It’s also important that you order your test cases in such a way that one follows the other. For example, for testing CRUD operations, you need to write the test cases in the order Create, Update and Delete.